C Programming Mock Test
This section presents you various set of Mock Tests related to C Programming Framework. You can download these sample mock tests at your local machine and solve offline at your convenience. Every mock test is supplied with a mock test key to let you verify the final score and grade yourself.
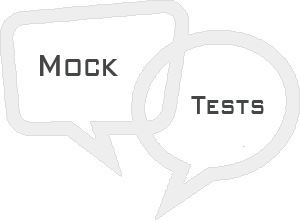
C Programming Mock Test I
Q 1 - What is the output of the following code snippet?
#include<stdio.h> main() { int const a = 5; a++; printf(“%d”,a); }
Answer : D
Explanation
Compile error - constant variable cannot be modified.
main() { int const a = 5; a++; printf(“%d”,a); }
Q 2 - What is the output of the following code snippet?
#include<stdio.h> main() { const int a = 5; a++; printf("%d", a); }
Answer : D
Explanation
Compile error - constant variable cannot be modified.
main() { const int a = 5; a++; printf("%d", a); }
Q 3 - What is the output of the below code snippet?
#include<stdio.h> main() { char s[]="hello", t[]="hello"; if(s==t){ printf("eqaul strings"); } }
Answer : C
Explanation
No output, as we are comparing both base addresses and they are not same.
Q 4 - What is the output of the below code snippet?
#include<stdio.h> main() { int a = 5, b = 3, c = 4; printf("a = %d, b = %d\n", a, b, c); }
Answer : A
Explanation
a=5,b=3 , as there are only two format specifiers for printing.
Q 5 - What is the output of the below code snippet?
#include<stdio.h> main() { int a = 1; float b = 1.3; double c; c = a + b; printf("%.2lf", c); }
Answer : A
Explanation
2.30, addition is valid and after decimal with is specified for 2 places.
Q 6 - What is the outpout of the following program?
#include<stdio.h> main() { enum { india, is=7, GREAT }; printf("%d %d", india, GREAT); }
Answer : C
Explanation
0 8, enums gives the sequence starting with 0. If assigned with a value the sequence continues from the assigned value.
Q 7 - What is the output of the following code snippet?
#include<stdio.h> main() { char c = 'A'+255; printf("%c", c); }
Answer : A
Explanation
A, the range of ASCII values for the ASCII characters is 0-255. Hence the addition operation circulates back to ‘A’
Q 8 - What is the output of the following code snippet?
#include<stdio.h> main() { short unsigned int i = 0; printf("%u\n", i--); }
Answer : A
Explanation
0, with post decrement operator value of the variable will be considered as the expression’s value and later gets decremented.
Q 9 - What is the output of the below code snippet?
#include<stdio.h> main() { unsigned x = 5, y=&x, *p = y+0; printf("%u",*p); }
Answer : D
Explanation
5, as p holds the address of x which is y+0
Q 10 - What is your comment on the below C statement?
signed int *p=(int*)malloc(sizeof(unsigned int));
C - Memory will be allocated but cannot hold an int value in the memory
Answer : D
Explanation
Option (d), as the size of int and unsigned is same, no problem in allocating memory.
Q 11 - What is the output of the following code snippet?
#include<stdio.h> main() { int x = 5; if(x==5) { if(x==5) break; printf("Hello"); } printf("Hi"); }
Answer : A
Explanation
compile error, keyword break can appear only within loop/switch statement.
Q 12 - What is the output of the following code snippet?
#include<stdio.h> main() { int x = 5; if(x=5) { if(x=5) break; printf("Hello"); } printf("Hi"); }
Answer : A
Explanation
compile error, keyword break can appear only within loop/switch statement.
Q 13 - What is the output of the following code snippet?
#include<stdio.h> main() { int x = 5; if(x=5) { if(x=5) printf("Hello"); } printf("Hi"); }
Answer : A
Explanation
HelloHi, both the if statement’s expression evaluates to be true.
Q 14 - What is the output of the below code snippet?
#include<stdio.h> main() { for(;;)printf("Hello"); }
Answer : A
Explanation
infinite loop, with second expression of ‘for’ being absent it is considered as true by default.
Q 15 - What is the output of the below code snippet?
#include<stdio.h> main() { for()printf("Hello"); }
Answer : D
Explanation
Compiler error, semi colons need to appear though the expressions are optional for the ‘for’ loop.
Q 16 - What is the output of the below code snippet?
#include<stdio.h> main() { for(1;2;3) printf("Hello"); }
Answer : A
Explanation
infinite loop, as the second expression is non-0, hence the condition is always true.
Answer : D
Explanation
-2, the one’s compliment of 1 is 1110 (binary) which is equivalent to two’s compliment of 2, ie -2.
Q 18 - What is the output of the following program?
#include<stdio.h> void f() { static int i; ++i; printf("%d", i); } main() { f(); f(); f(); }
Answer : D
Explanation
1 2 3, A static local variables retains its value between the function calls and the default value is 0.
Q 19 - What is the output of the following code snippet?
#include<stdio.h> main() { int *p = 15; printf("%d",*p); }
Answer : C
Explanation
Runtime error, as the pointer variable is not holding proper address, writing/reading the data from the same raises runtime error.
Q 20 - What is the output of the following program?
#include<stdio.h> main() { register int x = 5; int *p; p=&x; x++; printf("%d",*p); }
Answer : A
Explanation
Compile error, we cannot take the address of a register variable.
Q 21 - What is the output of the following program?
#include<stdio.h> main() { int x = 65, *p = &x; void *q=p; char *r=q; printf("%c",*r); }
Answer : B
Explanation
A, void pointer is a generic pointer and can hold any variable’s address. ASCII character for the value 65 is ‘A’
Q 22 - What is the output of the following program?
#include<stdio.h> void f() { printf(“Hello\n”); } main() { ; }
B - Error, as the function is not called.
C - Error, as the function is defined without its declaration
Answer : A
Explanation
No output, apart from the option (a) rest of the comments against the options are invalid.
Q 23 - What is the output of the following program?
#include<stdio.h> main() { printf("\"); }
Answer : D
Explanation
Compile error, Format string of printf is not terminated.
Q 24 - What is the output of the following program?
#include<stdio.h> { int x = 1; switch(x) { default: printf("Hello"); case 1: printf("hi"); break; } }
Answer : B
Explanation
Hi, control reaches default-case after comparing the rest of case constants.
Q 25 - What is the output of the following program?
#include<stdio.h> main() { struct { int x;} var = {5}, *p = &var; printf("%d %d %d",var.x,p->x,(*p).x); }
Answer : A
Explanation
5 5 5, the two possible ways of accessing structure elements using pointer is by using -> (arrow operator) OR *.
Q 26 - What is the output of the following program?
#include<stdio.h> void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x=5, y=3; swap(x,y); printf("%d %d", x, y); }
Answer : B
Explanation
5 3, call by value mechanism can’t alter actual arguments.
#include <stdio.h> void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x=5, y=3; swap(x,y); printf("%d %d", x, y); }
Q 27 - What will be printed for the below statement?
#include<stdio.h> main() { printf("%d",strcmp("strcmp()","strcmp()")); }
Answer : A
Explanation
0, strcmp return 0 if both the strings are equal
Q 28 - What is the following program doing?
#include<stdio.h> main() { FILE *stream=fopen("a.txt",'r'); }
A - Trying to open “a.txt” in read mode
B - Trying to open “a.txt” in write mode.
Answer : D
Explanation
Compile error, second argument for fopen is invalid, should be a string.
Q 29 - What is the output of the following program?
#include<stdio.h> main() { int r, x = 2; float y = 5; r = y%x; printf("%d", r); }
Answer : D
Explanation
Compile Error, It is invalid that either of the operands for the modulus operator (%) is a real number.
Q 30 - Which operator is used to continue the definition of macro in the next line?
Answer : D
Explanation
\, the first two are stringize and token pasting operators respectively. There is no such operator called $.
Q 31 - What is the size of the following union definition?
#include<stdio.h> union abc { char a,b,c,d,e,f,g,h; int i; }abc; main() { printf( "%d", sizeof( abc )); }
Answer : C
Explanation
union size is biggest element size of it. All the elements of the union share common memory.
Answer : D
Explanation
The size of ‘int’ depends upon the complier i.e. whether it is a 16 bit or 32 bit.
Q 33 - The type name/reserved word ‘short’ is ___
Answer : D
Explanation
short is used as an alternative of short int.
Q 34 - What is the value of ‘y’ for the following code snippet?
#include<stdio.h> main() { int x = 1; float y = x>>2; printf( "%f", y ); }
Answer : C
Explanation
0, data bits are lost for the above shift operation hence the value is 0.
Q 35 - What is the output of the following program?
#include<stdio.h> main() { float t = 2; switch(t) { case 2: printf("Hi"); default: printf("Hello"); } }
Answer : D
Explanation
Error, switch expression can’t be float value.
Q 36 - What is the output of the following program?
#include<stdio.h> main() { int i = 1; while(++i <= 5) printf("%d ",i++); }
Answer : B
Explanation
2 4, at while first incremented and later compared and in printf printed first and incremented later
Q 37 - What is the output of the following program?
#include<stdio.h> main() { int i = 1; while( i++<=5 ) printf("%d ",i++); }
Answer : C
Explanation
2 4 6, at while first compared and later incremented and in printf printed first and incremented later.
Q 38 - What is the output of the following program?
#include<stdio.h> main() { int i = 1; while(i++<=5); printf("%d ",i++); }
Answer : B
Explanation
6, there is an empty statement following ‘while’.
Q 39 - What is the output of the following program?
#include<stdio.h> main() { int x = 1; do printf("%d ", x); while(x++<=1); }
Answer : B
Explanation
1 2, do..while is an entry control loop. As the expression x++ is post form loop continues for 2nd time also.
Q 40 - What is the output of the following program?
#include<stdio.h> main() { int a[] = {1,2}, *p = a; printf("%d", p[1]); }
Answer : B
Explanation
2, as ‘p’ holds the base address then we can access array using ‘p’ just like with ‘a’
Q 41 - What is the output of the following program?
#include<stdio.h> main() { int a[3] = {2,1}; printf("%d", a[a[1]]); }
Answer : B
Explanation
1, The inner indirection evaluates to 1, and the value at index 1 for outer indirection is 1.
Q 42 - What is the output of the following program?
#include<stdio.h> main() { int a[3] = {2,,1}; printf("%d", a[a[0]]); }
Answer : D
Explanation
Compile error, invalid syntax in initializing the array.
Q 43 - What is the output of the following program?
#include<stdio.h> main() { int a[] = {2,1}; printf("%d", *a); }
Answer : C
Explanation
2, as ‘a’ refers to base address.
Q 44 - What is the output of the following program?
#include<stdio.h> main() { int i = 1; Charminar: printf("%d ",i++); if(i==3) break; if(i<=5) goto Charminar; }
Answer : D
Explanation
Compile error, wrong place for ‘break’ to appear.
Q 45 - What is the output of the following program?
#include<stdio.h> main() { int i = 13, j = 60; i ^= j; j ^= i; i ^= j; printf("%d %d", i, j); }
Answer : B
Explanation
60 13, its swapping.
Q 46 - What is the output of the following program?
#include<stdio.h> main() { union abc { int x; char ch; }var; var.ch = 'A'; printf("%d", var.x); }
Answer : C
Explanation
65, as the union variables share common memory for all its elements, x gets ‘A’ whose ASCII value is 65 and is printed.
Answer : C
Explanation
x, there is no such mode called “x”.
Q 48 - Function fopen() with the mode "r+" tries to open the file for __
Answer : A
Explanation
Option (a), the file should exist and opens for both reading & writing.
Q 49 - Identify the invalid constant used in fseek() function as ‘whence’ reference.
Answer : C
Explanation
SEEK_BEG, all the rest are valid constants defined in ‘stdio.h’
Q 50 - First operating system designed using C programming language.
Answer : C
Explanation
UNIX. C actually invented to write an operation system called UNIX. By 1973 the entire UNIX OS is designed using C.
Answer Sheet
Question Number | Answer Key |
---|---|
1 | D |
2 | D |
3 | C |
4 | A |
5 | A |
6 | C |
7 | A |
8 | A |
9 | D |
10 | D |
11 | A |
12 | A |
13 | A |
14 | A |
15 | D |
16 | A |
17 | D |
18 | D |
19 | C |
20 | A |
21 | B |
22 | A |
23 | D |
24 | B |
25 | A |
26 | B |
27 | A |
28 | D |
29 | D |
30 | D |
31 | C |
32 | D |
33 | D |
34 | C |
35 | D |
36 | B |
37 | C |
38 | B |
39 | B |
40 | B |
41 | B |
42 | D |
43 | C |
44 | D |
45 | B |
46 | C |
47 | C |
48 | A |
49 | C |
50 | C |