PHP - Ajax XML Parser
Advertisements
Ajax XML Example
Using with Ajax we can parser xml from local directory as well as servers, Below example demonstrate how to parser xml with web browser.
<html> <head> <script> function showCD(str) { if (str == "") { document.getElementById("txtHint").innerHTML = ""; return; } if (window.XMLHttpRequest) { // code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp = new XMLHttpRequest(); }else { // code for IE6, IE5 xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange = function() { if (xmlhttp.readyState == 4 && xmlhttp.status == 200) { document.getElementById("txtHint").innerHTML = xmlhttp.responseText; } } xmlhttp.open("GET","getcourse.php?q="+str,true); xmlhttp.send(); } </script> </head> <body> <form> Select a Course: <select name = "cds" onchange = "showCD(this.value)"> <option value = "">Select a course:</option> <option value = "Android">Android </option> <option value = "Html">HTML</option> <option value = "Java">Java</option> <option value = "Microsoft">MS technologies</option> </select> </form> <div id = "txtHint"><b>Course info will be listed here...</b></div> </body> </html>
The above example will call getcourse.php using with GET method. getcourse.php file loads catalog.xml. getcourse.php is as shown below −
<?php $q = $_GET["q"]; $xmlDoc = new DOMDocument(); $xmlDoc->load("catalog.xml"); $x = $xmlDoc->getElementsByTagName('COURSE'); for ($i = 0; $i<=$x->length-1; $i++) { = if ($x->item($i)->nodeType == 1) { if ($x->item($i)->childNodes->item(0)->nodeValue == $q) { $y = ($x->item($i)->parentNode); } } } $cd = ($y->childNodes); for ($i = 0;$i<$cd->length;$i++) { if ($cd->item($i)->nodeType == 1) { echo("<b>" . $cd->item($i)->nodeName . ":</b> "); echo($cd->item($i)->childNodes->item(0)->nodeValue); echo("<br>"); } } ?>
Catalog.xml
XML file having list of courses and details.This file is accessed by getcourse.php
<CATALOG> <SUBJECT> <COURSE>Android</COURSE> <COUNTRY>India</COUNTRY> <COMPANY>TutorialsPoint</COMPANY> <PRICE>$10</PRICE> <YEAR>2015</YEAR> </SUBJECT> <SUBJECT> <COURSE>Html</COURSE> <COUNTRY>India</COUNTRY> <COMPANY>TutorialsPoint</COMPANY> <PRICE>$15</PRICE> <YEAR>2015</YEAR> </SUBJECT> <SUBJECT> <COURSE>Java</COURSE> <COUNTRY>India</COUNTRY> <COMPANY>TutorialsPoint</COMPANY> <PRICE>$20</PRICE> <YEAR>2015</YEAR> </SUBJECT> <SUBJECT> <COURSE>Microsoft</COURSE> <COUNTRY>India</COUNTRY> <COMPANY>TutorialsPoint</COMPANY> <PRICE>$25</PRICE> <YEAR>2015</YEAR> </SUBJECT> </CATALOG>
It will produce the following result −
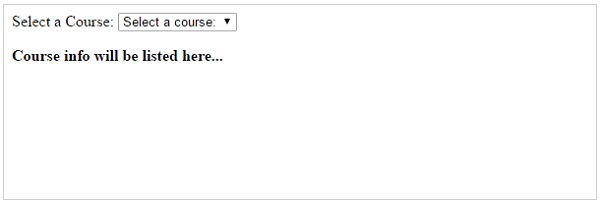
Advertisements