JScript Web Resources
JScript Web Resources are probably the most important type of web resources that you will be using with Microsoft Dynamics CRM.
Applications of JavaScript in CRM
Form Event Programming
Form Event Programming is used to handle client-side behaviors such as what happens when a user opens a form, changes some data, moves through tabs, etc. To achieve such client-side interactions you will be writing JavaScript code and adding it as a JScript Web Resource in CRM. However, the JavaScript code which you will write has to use Dynamic CRM’s Xrm.Page model and not the standard JavaScript DOM. Using Xrm.Page model is Microsoft’s way of coding which ensures that any code you write using this model will be compatible with any future versions of CRM.
Web Resources
In addition to being used in Form Event Programming, JavaScript is used in other applications of CRM such as −
Open Forms, Views and Dialogs with a unique URL.
Using OData and SOAP endpoints to interact with web services.
Referencing JavaScript code inside other Web Resources (such as HTML web resources).
In such cases, you would write your JavaScript code (using Xrm.Page model) and add it as a JScript Web Resource in CRM, which can then be referenced anywhere with a unique URI.
Ribbon Customizations
Finally, one of the other common use of JavaScript is to handle ribbon customizations such as −
- Display/Hide ribbon buttons based on some logic
- Enable/Disable ribbon buttons based on some logic
- Handle what happens when you click a certain ribbon button
To handle such scenarios, you will write your JavaScript logic (using Xrm.Page model) and then add it as a JScript Web Resource. This Web Resource can then be referenced in the ribbon button’s XML and we can specify which method in which JScript file to call to check if a ribbon button should be displayed/hidden or enabled/disabled or handle click events.
Xrm.Page Object Model
Following is the Xrm.Page object’s hierarchy showing the available namespaces, objects, and their collections. You will be using these properties while writing JScript code.
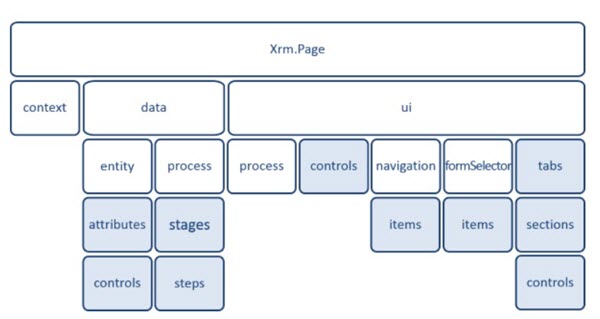
Namespaces
Sr.No | Object & Description |
---|---|
1 | Context Provides methods to retrieve context-specific information such as organization details, logged-in user details, or parameters that were passed to the form in a query string. |
2 | Data Provides access to the entity data and methods to manage the data in the form as well as in the business process flow control. |
3 | UI Contains methods to retrieve information about the user interface, in addition to collections for several sub-components of the form. |
Objects
Sr.No | Object & Description |
---|---|
1 | Entity Provides method to −
|
2 | Process Methods to retrieve properties of business process flow. |
3 | Navigation Provides access to navigation items using items collection. |
4 | FormSelector Uses Items collection to access available forms to the user. Also uses the navigation method to close and open forms. |
5 | Stages Each process has a collection of stages that can be accessed using getStages method of process. |
6 | Steps Each stage comprises of various steps that can be accessed using getSteps method of stage. |
Collections
Sr.No | Collections & Description |
---|---|
1 | Attributes Provides access to entity attributes available on the form. |
2 | Controls ui.controls − Provides access to each control present on the form. attribute.controls − Provides access to all the controls within an attribute. section.controls − Provides access to all the controls within a section. |
3 | Items Provides access to all the navigation items on a form. |
4 | Tabs Provides access to all the tabs on a form. |
5 | Sections Provides access to all the sections on a form. |
Supported Events in Form Programming
The Form Programming using Xrm.Page model allows you to handle the following form events −
- onLoad
- onSave
- onChange
- TabStateChange
- OnReadyStateComplete
- PreSearch
- Business Process Flow control events
Form Programming Example
In this example, we will put some validations on the Contact form based on the PreferredMethodofCommunication that the user selects. Hence, if the user selects his/her preferred method as Email, then the Email field should become mandatory and similarly for other fields of Phone and Fax.
Step 1 − Create a JavaScript file named contacts.js and copy the following code.
function validatePreferredMethodOfCommunication() { //get the value of Preffered Method of Communication code var prefferedContactMethodCode = Xrm.Page.getAttribute('preferredcontactmetho dcode').getValue(); //if Preferred Method = Any, make all fields as non-mandatory //else if Preferred Method = Phone, make Mobile Phone field mandatory //and all other fields as non-mandatory //else if Preferred Method = Fax, make Fax field mandatory //and all other fields as non-mandatory if(prefferedContactMethodCode == 1) { clearAllMandatoryFields(); } if(prefferedContactMethodCode == 2) { clearAllMandatoryFields(); Xrm.Page.getAttribute('emailaddress1').setRequiredLevel('required'); } else if(prefferedContactMethodCode == 3) { clearAllMandatoryFields(); Xrm.Page.getAttribute('mobilephone').setRequiredLevel('required'); } else if(prefferedContactMethodCode == 4) { clearAllMandatoryFields(); Xrm.Page.getAttribute('fax').setRequiredLevel('required'); } } function clearAllMandatoryFields() { //clear all mandatory fields Xrm.Page.getAttribute('emailaddress1').setRequiredLevel('none'); Xrm.Page.getAttribute('mobilephone').setRequiredLevel('none'); Xrm.Page.getAttribute('fax').setRequiredLevel('none'); }
Step 2 − Open the Contact entity form by navigating to Settings → Customizations → Customize the System → Contact entity → Forms → Main Form.
Step 3 − Click Form Properties.
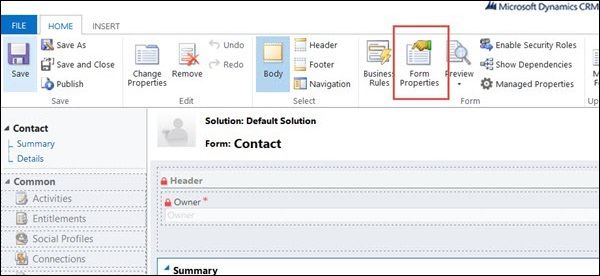
Step 4 − From the Form Properties window, click Add.
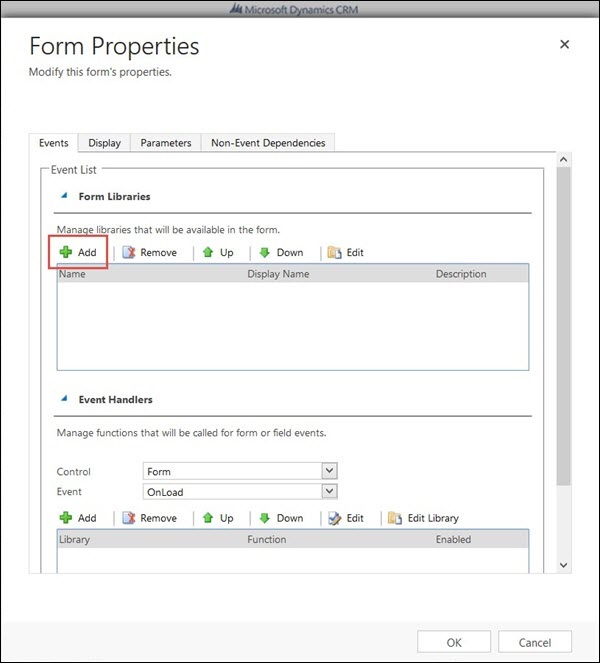
Step 5 − In the next Look Up Web Resource Record window, click New since we are creating a new web resource.
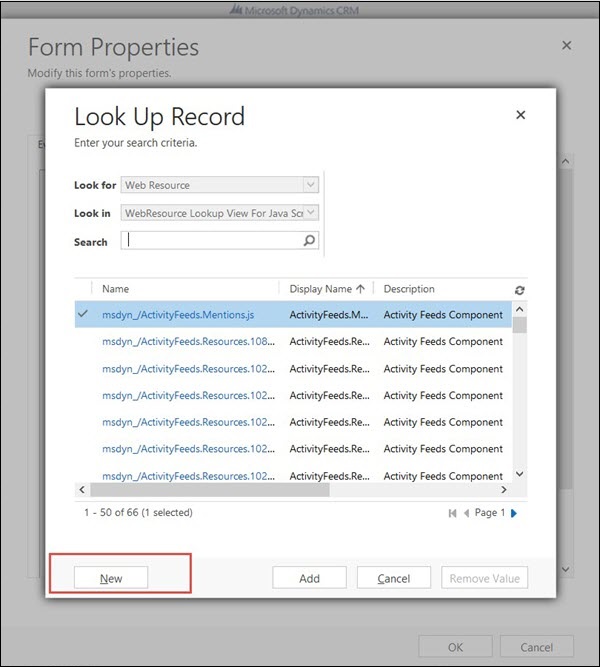
Step 6 − In the New Web Resource window, enter the following details −
Name − new_contacts.js
Display Name − contacts.js
Type − JScript
Upload File − Upload the JavaScript file that you created from your local machine.
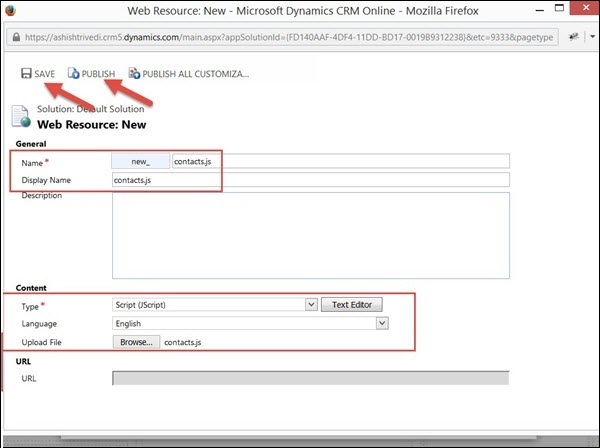
Step 7 − Click Save followed by Publish. After this close the window and you will be back to Look Up Web Resource Record window.
Step 8 − Here, you can now see the new_contacts.js web resource. Select it and click Add. You have now successfully added a new web resource and registered it on the form.
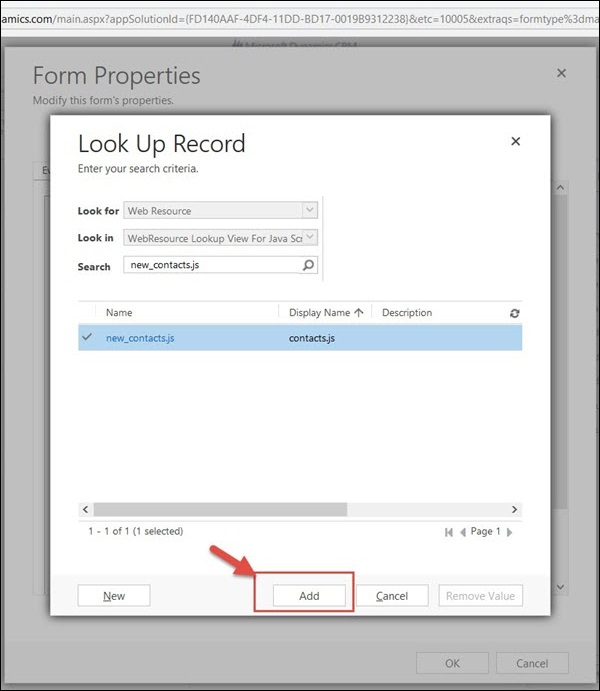
Step 9 − Now we will add an event handler on the change of Preferred Method of Communication field. This event handler will call the JavaScript function that we just wrote. Select the following options from the Event Handler section.
Control − Preferred Method of Communication
Event − OnChange
Then, click the Add button, as shown in the following screenshot.
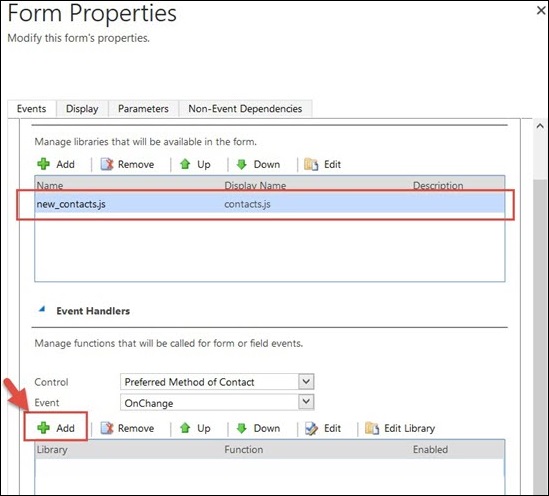
Step 10 − In the next window of Handler Properties, we will specify the method to be called when the change event occurs.
Select Library as new_contacts.js and Function as validatePreferredMethodOfCommunication. Click OK.
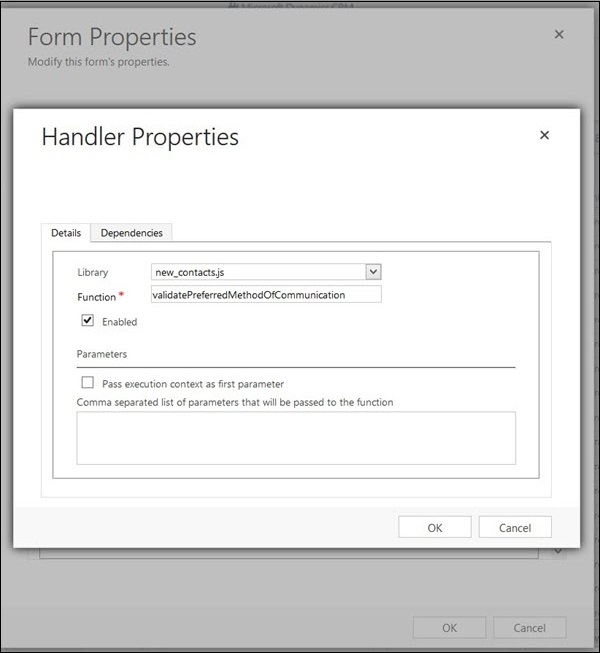
Step 11 − You will now be able to see the Form Library (Web Resource) and events registered on it. Click OK.
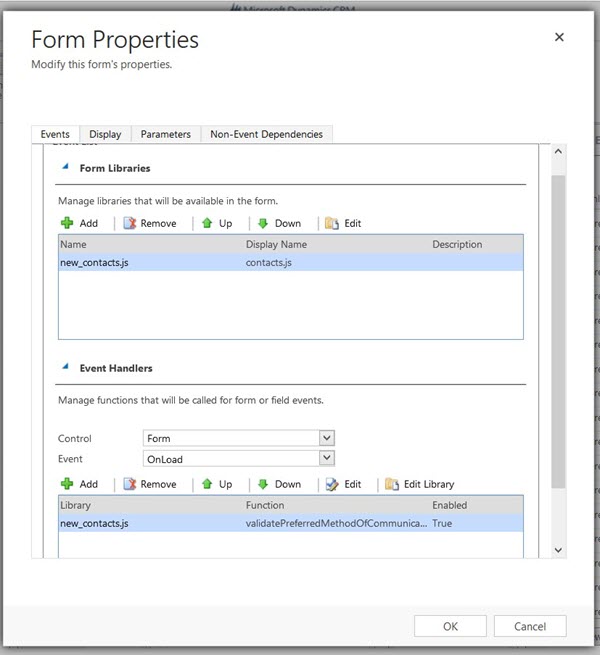
Step 12 − Click Save followed by Publish.
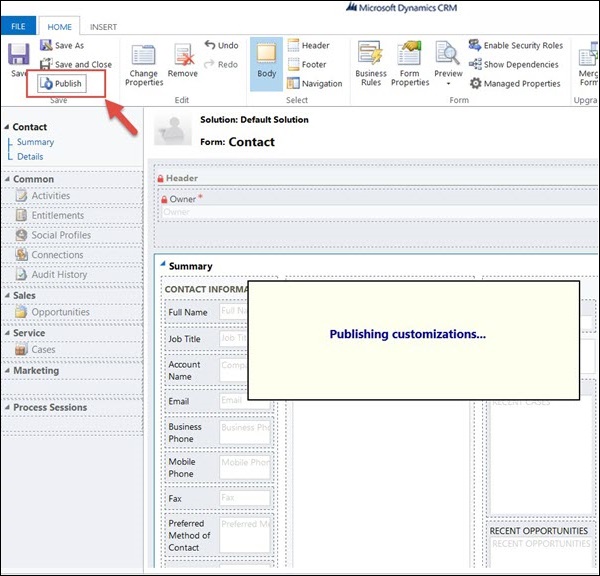
Step 13 − Now open any Contact form and set the Preferred Method of Communication as Phone. This will make the Mobile Phone field as mandatory. If you now try to save this contact without entering any mobile number, it will give you an error saying ‘You must provide a value for Mobile Phone’.
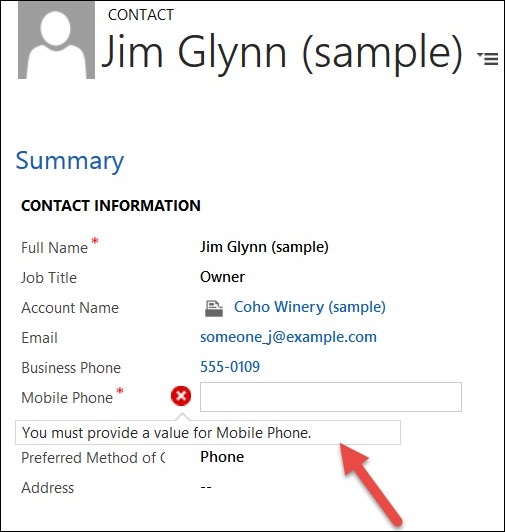
Conclusion
In this chapter, we started by understanding the three important applications of JavaScript in CRM. Later, we explored the Xrm.Page model and used it to learn Form programming along with an example.