Microsoft Dynamics CRM - Web Services
Microsoft Dynamics CRM provides two important web services that are used to access CRM from an external application and invoke web methods to perform common business data operations such as create, delete, update, and find in CRM.
Consider the following scenarios −
You have an external .NET application, which needs to talk to CRM. For example, you may want to insert a Contact record in CRM when a new customer is registered in your external application.
Or maybe, you want to search records in CRM and display the search results in your external application.
In such scenarios, you can use the web services exposed by CRM to consume them in your application and perform create, delete, update, and find operations in CRM.
IDiscoveryService Web Service
This web service returns a list of organizations that the specified user belongs to and the URL endpoint for each of the organization.
IOrganizationService Web Service
This web service is the primary web service used for accessing data and metadata in CRM. The IOrganizationService uses two important assemblies –Microsoft.Xrm.Sdk.dll and Microsoft.Crm.Sdk.Proxy.dll. These assemblies can be found in the CRM SDK package inside the Bin folder.
Microsoft.Xrm.Sdk.dll
This assembly defines the core xRM methods and types, including proxy classes to make the connection to Microsoft Dynamics CRM simpler, authentication methods, and the service contracts.
Microsoft.Crm.Sdk.Proxy.dll
This assembly defines the requests and responses for non-core messages as well as enumerations required for working with the organization data. Following are the namespaces supported by these two assemblies.
Each of these assemblies support certain messages, which will be used to work with the data stored in any entity. A complete list of messages supported by them can be found in the following links −
Supported xRM Messages − https://msdn.microsoft.com/en-us/library/gg334698.aspx
Supported CRM Messages − https://msdn.microsoft.com/en-us/library/gg309482.aspx
IOrganizationService Web Service Methods
The IOrganizationService provides eight methods that allows you to perform all the common operations on the system and custom entities as well as organization metadata.
Sr.No | Method & Description |
---|---|
1 | IOrganizationService.Create Creates a record. |
2 | IOrganizationService.Update Updates an existing record. |
3 | IOrganizationService. Retrieve Retrieves a record. |
4 | IOrganizationService. RetrieveMultiple Retrieves a collection of records. |
5 | IOrganizationService. Delete Deletes a record. |
6 | IOrganizationService. Associate Creates a link between records. |
7 | IOrganizationService.Disassociate Deletes a link between records. |
8 | IOrganizationService.Execute Used for common record processing as well as specialized processing such as case resolution, duplicate detection, etc. |
Web Service Example
To understand how the web services work in CRM, we will look at an example provided by CRM SDK. In this example, we will create a new Account record, update it, and then finally delete it using the CRM IOrganizationService web service.
Step 1 − Open the folder where you had extracted CRM SDK. Now open the QuickStartCS.sln solution by browsing to the following location:SDK\SampleCode\CS\QuickStart
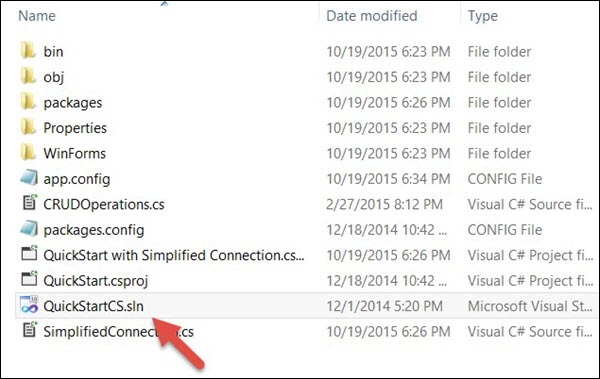
Step 2 − We will be exploring the QuickStart with Simplified Connection project. Open app.config in this project. By default, the connectionStrings section in this file will be commented.
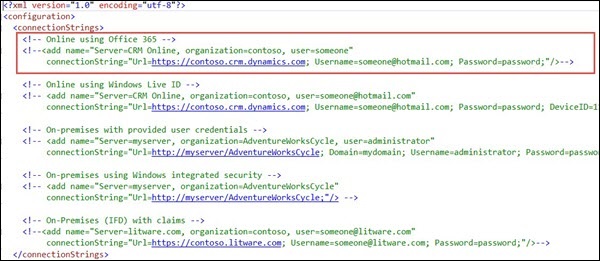
From this, uncomment the first connection string key and edit the following three details −
Url − Specify the URL of your CRM instance. In our case, since we are using the online version of CRM, you will have to mention that URL.
Username − Your CRM Online user name.
Password − Your CRM Online password.
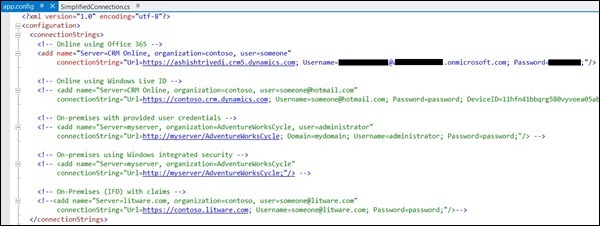
Step 3 − Open the SimplifiedConnection.cs file in this project and Runmethod inside it.
public void Run(StringconnectionString, boolpromptforDelete) { try { // Establish a connection to the organization web service using CrmConnection. Microsoft.Xrm.Client.CrmConnection connection = CrmConnection.Parse(connectionString); // Obtain an organization service proxy. // The using statement assures that the service proxy will be properly disposed. using(_orgService = new OrganizationService(connection)) { //Create any entity records this sample requires. CreateRequiredRecords(); // Obtain information about the logged on user from the web service. Guid userid = ((WhoAmIResponse)_orgService.Execute(new WhoAmIRequest())).UserId; SystemUser systemUser = (SystemUser)_orgService.Retrieve("systemuser",userid, new ColumnSet(newstring[]{"firstname","lastname"})); Console.WriteLine("Logged on user is {0} {1}.", systemUser.FirstName,systemUser.LastName); // Retrieve the version of Microsoft Dynamics CRM. RetrieveVersionRequest versionRequest = new RetrieveVersionRequest(); RetrieveVersionResponse versionResponse = (RetrieveVersionResponse)_orgService.Execute(versionRequest); Console.WriteLine("Microsoft Dynamics CRM version {0}.", versionResponse.Version); // Instantiate an account object. Note the use of option set enumerations defined in OptionSets.cs. // Refer to the Entity Metadata topic in the SDK documentation to determine which attributes must // be set for each entity. Account account = new Account{Name = "Fourth Coffee"}; account.AccountCategoryCode = new OptionSetValue( (int)AccountAccountCateg oryCode.PreferredCustomer); account.CustomerTypeCode = new OptionSetValue( (int)AccountCustomerTypeCod e.Investor); // Create an account record named Fourth Coffee. _accountId = _orgService.Create(account); Console.Write("{0} {1} created, ",account.LogicalName,account.Name); // Retrieve the several attributes from the new account. ColumnSet cols = new ColumnSet( new String[]{"name","address1_postalcode","lastusedincampaign"}); Account retrievedAccount = (Account)_orgService.Retrieve("account", _accountId, cols); Console.Write("retrieved, "); // Update the postal code attribute. retrievedAccount.Address1_PostalCode = "98052"; // The address 2 postal code was set accidentally, so set it to null. retrievedAccount.Address2_PostalCode = null; // Shows use of a Money value. retrievedAccount.Revenue = new Money(5000000); // Shows use of a Boolean value. retrievedAccount.CreditOnHold = false; // Update the account record. _orgService.Update(retrievedAccount); Console.WriteLine("and updated."); // Delete any entity records this sample created. DeleteRequiredRecords(promptforDelete); } } // Catch any service fault exceptions that Microsoft Dynamics CRM throws. catch(FaultException<microsoft.xrm.sdk.organizationservicefault>) { // You can handle an exception here or pass it back to the calling method. throw; } }
Step 4 − This method basically demonstrates all the CRUD operations using CRM web services. The code first creates an organization instance, then creates an Account record, updates the created record and then finally deletes it. Let us look at the important components of this code. To see on-the-go changes in CRM when this code runs, you can debug this code step-by-step (as we discuss below) and simultaneously see the changes in CRM.
Step 4.1 − Establishes the connection to the organization using the connection string that we had modified in Step 2.
Microsoft.Xrm.Client.CrmConnection connection = CrmConnection.Parse(connectionString);
Step 4.2 − Obtains a proxy instance of CRM organization web service.
_orgService = new OrganizationService(connection)
Step 4.3 − Creates a new Account entity object and sets its Name, AccountCategoryCode and CustomerTypeCode.
Account account = new Account{Name = "Fifth Coffee"}; account.AccountCategoryCode = new OptionSetValue( (int)AccountAccountCategoryCode.P referredCustomer); account.CustomerTypeCode = new OptionSetValue( (int)AccountCustomerTypeCode.Investor);
Step 4.4 − Creates the new record using the Create method of organization service.
_accountId = _orgService.Create(account);
If you navigate to CRM, you will see a newly created account record.
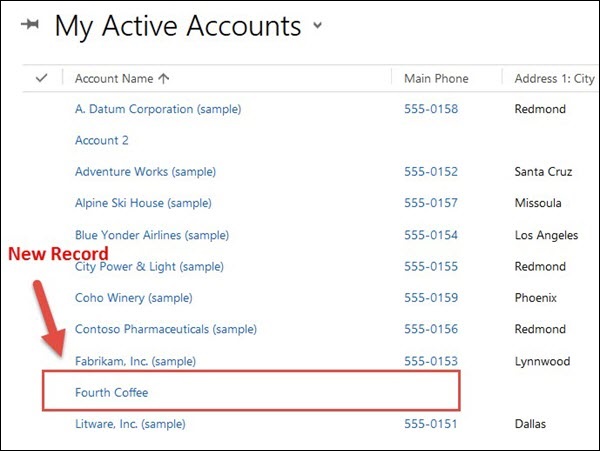
Step 4.5 − Once the account gets created, the service retrieves back the record from CRM using Retrieve web service method.
ColumnSet cols = new ColumnSet(new String[]{ "name","address1_postalcode","lastusedincampaign"}); Account retrievedAccount = (Account)_orgService.Retrieve("account", _accountId, cols);
Step 4.6 − Once you have the retrieved record, you can set the updated value of the record.
retrievedAccount.Address1_PostalCode = "98052"; retrievedAccount.Address2_PostalCode = null; retrievedAccount.Revenue = new Money(5000000); retrievedAccount.CreditOnHold = false;
Step 4.7 − After setting the updated value of the record, update the record back to CRM database using the Update web service method.
_orgService.Update(retrievedAccount);
If you open the record in CRM, you will see these values updated there.
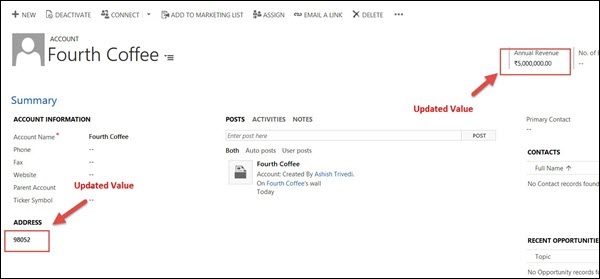
Step 4.8 − Finally, delete the record using the Delete web service method.
_orgService.Delete(Account.EntityLogicalName, _accountId);
If you now refresh the same record in CRM, you will see that the record is no more available since it is already deleted.
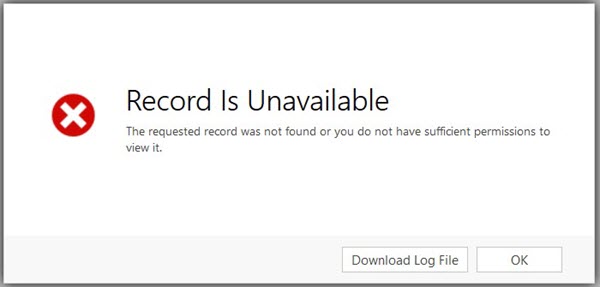
Conclusion
In this chapter, we dealt with two important web services provided by CRM and a working example of how these web services can be used from an external application to perform various CRUD operations.