Xamarin - Gallery
A Gallery is a type of view that is used to show items in a horizontal scrollable list. The selected item is then shown at the center. In this example, you are going to create a gallery containing images which are scrollable horizontally. An image when clicked will display a number for the selected image.
First of all, create a new project and give it a name, e.g., Gallery App Tutorial. Before you start to code, paste 7 images into the resource /drawable folder. Navigate to main.axml under resources folder and a gallery in between the linear layout tags.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="https://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#d3d3d3"> <Gallery android:id="@+id/gallery" android:layout_width="fill_parent" android:layout_height="wrap_content" android:padding="10dp" /> </LinearLayout>
Create a new class called ImageAdapter. This class is going to be used to bind the images to the gallery we created above.
The first step is to add a class that contains a context cont which we use to store fields.
public class ImageAdapter : BaseAdapter { Context cont; public ImageAdapter(Context ct) { cont = ct; } }
Next, we count the array list which contains our image and returns its size.
public override int Count { get { return imageArraylist.Length; } }
In the next step, we get the position of the item. The following code shows how to do it.
public override Java.Lang.Object GetItem(int position) { return null; } public override long GetItemId(int position) { return 0; }
In the next step, we create an imageview for the items referenced by the adapter.
public override View GetView(int position,View convertView, ViewGroup parent) { ImageView img = new ImageView(cont); img.SetImageResource(imageArraylist[position]); img.SetScaleType(ImageView.ScaleType.FitXy); img.LayoutParameters = new Gallery.LayoutParams(200, 100); return img; }
In the final step, we create a reference to the images we added in the resources.drawable folder. To do this, we create an array to hold the collection of images. The following code explains how to do it.
int[] imageArraylist = { Resource.Drawable.img1, Resource.Drawable.img2, Resource.Drawable.img3, Resource.Drawable.img4, Resource.Drawable.img5, Resource.Drawable.img6, }; }
Next, we go to mainActivity.cs and insert the following code under the OnCreate() method.
Gallery myGallery = (Gallery)FindViewById<Gallery>(Resource.Id.gallery); myGallery.Adapter = new ImageAdapter(this); myGallery.ItemClick += delegate(object sender, AdapterView.ItemClickEventArgs args) { Toast.MakeText(this, args.Position.ToString(), ToastLength.Short).Show(); }
Finally, build and run your application to view the output.
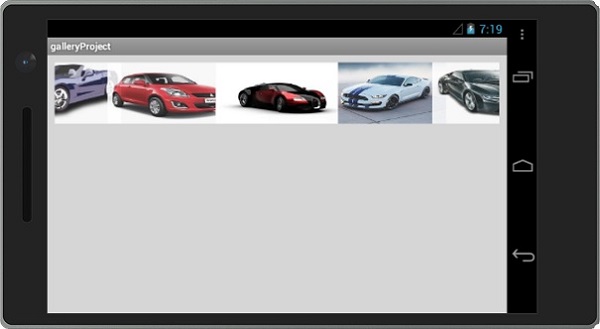