Ionic - Cordova Camera
The Cordova camera plugin uses the native camera for taking pictures or getting images from the image gallery.
Using Camera
Open your project root folder in command prompt, then download and install the Cordova camera plugin with the following command.
C:\Users\Username\Desktop\MyApp> cordova plugin add org.apache.cordova.camera
Now, we will create a service for using a camera plugin. We will use the AngularJS factory and promise object $q that needs to be injected to the factory.
services.js Code
.factory('Camera', function($q) { return { getPicture: function(options) { var q = $q.defer(); navigator.camera.getPicture(function(result) { q.resolve(result); }, function(err) { q.reject(err); }, options); return q.promise; } } });
To use this service in the app, we need to inject it to a controller as a dependency. Cordova camera API provides the getPicture method, which is used for taking photos using a native camera.
The native camera settings can be additionally customized by passing the options parameter to the takePicture function. Copy the above-mentioned code sample to your controller to trigger this behavior. It will open the camera application and return a success callback function with the image data or error callback function with an error message. We will also need two buttons that will call the functions we are about to create and we need to show the image on the screen.
HTML Code
<button class = "button" ng-click = "takePicture()">Take Picture</button> <button class = "button" ng-click = "getPicture()">Open Gallery</button> <img ng-src = "{{user.picture}}">
Controller Code
.controller('MyCtrl', function($scope, Camera) { $scope.takePicture = function (options) { var options = { quality : 75, targetWidth: 200, targetHeight: 200, sourceType: 1 }; Camera.getPicture(options).then(function(imageData) { $scope.picture = imageData;; }, function(err) { console.log(err); }); }; })
The output will look as shown in the following screenshot.
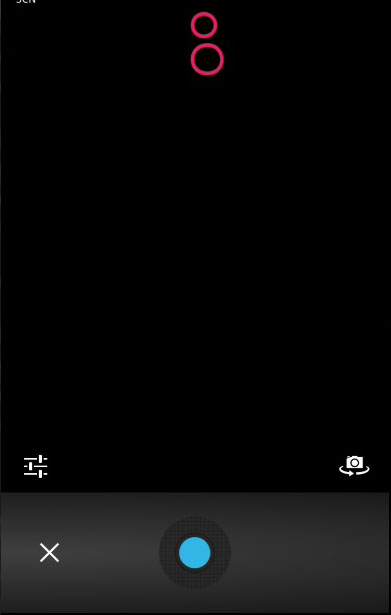
If you want to use images from your gallery, the only thing you need to change is the sourceType method from your options parameter. This change will open a dialog popup instead of camera and allow you to choose the image you want from the device.
You can see the following code, where the sourceType option is changed to 0. Now, when you tap the second button, it will open the file menu from the device.
Controller Code
.controller('MyCtrl', function($scope, Camera) { $scope.getPicture = function (options) { var options = { quality : 75, targetWidth: 200, targetHeight: 200, sourceType: 0 }; Camera.getPicture(options).then(function(imageData) { $scope.picture = imageData;; }, function(err) { console.log(err); }); }; })
The output will look as shown in the following screenshot.

When you save the image you took, it will appear on the screen. You can style it the way you want.

Several other options can be used as well, some of which are given in the following table.
Parameter | Type | Details |
---|---|---|
quality | Number | The quality of the image, range 0-100 |
destinationType | Number | Format of the image. |
sourceType | Number | Used for setting the source of the picture. |
allowEdit | boolean | Used for allowing editing of the image. |
encodingType | Number | Value 0 will set JPEG, and value 1 will set PNG. |
targetWidth | Number | Used for scaling image width. |
targetHeight | Number | Used for scaling image height. |
mediaType | string | Used for setting the media type. |
cameraDirection | Number | Value 0 will set the back camera, and value 1 will set the front camera. |
popoverOptions | string | IOS-only options that specify popover location in iPad. |
saveToPhotoAlbum | boolean | Used for saving image to photo album. |
correctOrientation | boolean | Used for correcting orientation of the captured images. |